Chào ace, bài này chúng ta sẽ tìm hiểu về một trong các thuật toán sắp xếp được sử dụng nhiều trong lập trình và thực tế nhất đó là Bubble Sort, sau đây cafedev sẽ giới thiệu và chia sẻ chi tiết(khái niệm, ứng dụng của nó, code ví dụ, điểm mạnh, điểm yếu…) về Bubble Sort thông qua các phần sau.
Nội dung chính
1. Giới thiệu
Bubble Sort là thuật toán sắp xếp đơn giản nhất hoạt động bằng cách hoán đổi nhiều lần các phần tử liền kề nếu chúng sai thứ tự.
Ví dụ dùng thuật toán Bubble Sort:
Vòng chạy đầu tiên:
(5 1 4 2 8) -> (1 5 4 2 8), Ở đây, thuật toán so sánh hai phần tử đầu tiên và hoán đổi vì 5> 1.
(1 5 4 2 8) -> (1 4 5 2 8), Hoán đổi vì 5> 4
(1 4 5 2 8) -> (1 4 2 5 8), Hoán đổi vì 5> 2
(1 4 2 5 8) -> (1 4 2 5 8), Bây giờ, vì các phần tử này đã có thứ tự (8> 5), thuật toán không hoán đổi chúng.
Vòng chạy thứ hai:
(1 4 2 5 8) -> (1 4 2 5 8)
(1 4 2 5 8) -> (1 2 4 5 8), Hoán đổi vì 4> 2
(1 2 4 5 8) -> (1 2 4 5 8)
(1 2 4 5 8) -> (1 2 4 5 8)
Bây giờ, mảng đã được sắp xếp, nhưng thuật toán của chúng tôi không biết liệu nó có hoàn thành hay không. Thuật toán cần một lần chuyển toàn bộ mà không có bất kỳ sự hoán đổi nào để biết nó được sắp xếp.
Vòng chạy thứ ba:
(1 2 4 5 8) -> (1 2 4 5 8)
(1 2 4 5 8) -> (1 2 4 5 8)
(1 2 4 5 8) -> (1 2 4 5 8)
(1 2 4 5 8) -> (1 2 4 5 8)
2. Code ví dụ trên nhiều ngôn ngữ lập trình
C++
// C++ program for implementation of Bubble sort
#include <bits/stdc++.h>
using namespace std;
void swap(int *xp, int *yp)
{
int temp = *xp;
*xp = *yp;
*yp = temp;
}
// A function to implement bubble sort
void bubbleSort(int arr[], int n)
{
int i, j;
for (i = 0; i < n-1; i++)
// Last i elements are already in place
for (j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
swap(&arr[j], &arr[j+1]);
}
/* Function to print an array */
void printArray(int arr[], int size)
{
int i;
for (i = 0; i < size; i++)
cout << arr[i] << " ";
cout << endl;
}
// Driver code
int main()
{
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
cout<<"Sorted array: \n";
printArray(arr, n);
return 0;
}
C
// C program for implementation of Bubble sort
#include <stdio.h>
void swap(int *xp, int *yp)
{
int temp = *xp;
*xp = *yp;
*yp = temp;
}
// A function to implement bubble sort
void bubbleSort(int arr[], int n)
{
int i, j;
for (i = 0; i < n-1; i++)
// Last i elements are already in place
for (j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
swap(&arr[j], &arr[j+1]);
}
/* Function to print an array */
void printArray(int arr[], int size)
{
int i;
for (i=0; i < size; i++)
printf("%d ", arr[i]);
printf("\n");
}
// Driver program to test above functions
int main()
{
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
printf("Sorted array: \n");
printArray(arr, n);
return 0;
}
Java
// Java program for implementation of Bubble Sort
class BubbleSort
{
void bubbleSort(int arr[])
{
int n = arr.length;
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
{
// swap arr[j+1] and arr[j]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
/* Prints the array */
void printArray(int arr[])
{
int n = arr.length;
for (int i=0; i<n; ++i)
System.out.print(arr[i] + " ");
System.out.println();
}
// Driver method to test above
public static void main(String args[])
{
BubbleSort ob = new BubbleSort();
int arr[] = {64, 34, 25, 12, 22, 11, 90};
ob.bubbleSort(arr);
System.out.println("Sorted array");
ob.printArray(arr);
}
}
Python
# Python program for implementation of Bubble Sort
def bubbleSort(arr):
n = len(arr)
# Traverse through all array elements
for i in range(n):
# Last i elements are already in place
for j in range(0, n-i-1):
# traverse the array from 0 to n-i-1
# Swap if the element found is greater
# than the next element
if arr[j] > arr[j+1] :
arr[j], arr[j+1] = arr[j+1], arr[j]
# Driver code to test above
arr = [64, 34, 25, 12, 22, 11, 90]
bubbleSort(arr)
print ("Sorted array is:")
for i in range(len(arr)):
print ("%d" %arr[i]),
C#
// C# program for implementation
// of Bubble Sort
using System;
class GFG
{
static void bubbleSort(int []arr)
{
int n = arr.Length;
for (int i = 0; i < n - 1; i++)
for (int j = 0; j < n - i - 1; j++)
if (arr[j] > arr[j + 1])
{
// swap temp and arr[i]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
/* Prints the array */
static void printArray(int []arr)
{
int n = arr.Length;
for (int i = 0; i < n; ++i)
Console.Write(arr[i] + " ");
Console.WriteLine();
}
// Driver method
public static void Main()
{
int []arr = {64, 34, 25, 12, 22, 11, 90};
bubbleSort(arr);
Console.WriteLine("Sorted array");
printArray(arr);
}
}
PHP
<?php
// PHP program for implementation
// of Bubble Sort
function bubbleSort(&$arr)
{
$n = sizeof($arr);
// Traverse through all array elements
for($i = 0; $i < $n; $i++)
{
// Last i elements are already in place
for ($j = 0; $j < $n - $i - 1; $j++)
{
// traverse the array from 0 to n-i-1
// Swap if the element found is greater
// than the next element
if ($arr[$j] > $arr[$j+1])
{
$t = $arr[$j];
$arr[$j] = $arr[$j+1];
$arr[$j+1] = $t;
}
}
}
}
// Driver code to test above
$arr = array(64, 34, 25, 12, 22, 11, 90);
$len = sizeof($arr);
bubbleSort($arr);
echo "Sorted array : \n";
for ($i = 0; $i < $len; $i++)
echo $arr[$i]." ";
?>
Kết quả:
Sorted array:
11 12 22 25 34 64 90
Hình minh họa thuật toán Bubble Sort chạy.
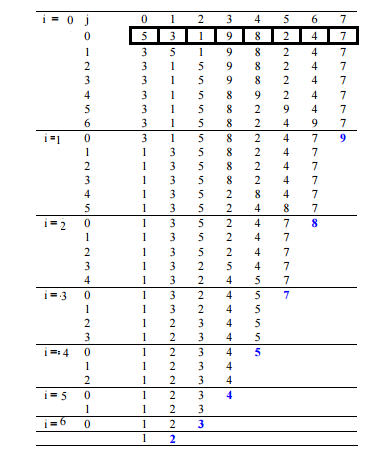
Cách triển khai tối ưu hoá hơn:
Hàm trên luôn chạy O (n ^ 2) thời gian ngay cả khi mảng được sắp xếp. Nó có thể được tối ưu hóa bằng cách dừng thuật toán nếu vòng lặp bên trong không gây ra bất kỳ sự hoán đổi nào.
Trên C++
// Optimized implementation of Bubble sort
#include <stdio.h>
void swap(int *xp, int *yp)
{
int temp = *xp;
*xp = *yp;
*yp = temp;
}
// An optimized version of Bubble Sort
void bubbleSort(int arr[], int n)
{
int i, j;
bool swapped;
for (i = 0; i < n-1; i++)
{
swapped = false;
for (j = 0; j < n-i-1; j++)
{
if (arr[j] > arr[j+1])
{
swap(&arr[j], &arr[j+1]);
swapped = true;
}
}
// IF no two elements were swapped by inner loop, then break
if (swapped == false)
break;
}
}
/* Function to print an array */
void printArray(int arr[], int size)
{
int i;
for (i=0; i < size; i++)
printf("%d ", arr[i]);
printf("n");
}
// Driver program to test above functions
int main()
{
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
printf("Sorted array: \n");
printArray(arr, n);
return 0;
}
Trên Java
// Optimized java implementation
// of Bubble sort
import java.io.*;
class GFG
{
// An optimized version of Bubble Sort
static void bubbleSort(int arr[], int n)
{
int i, j, temp;
boolean swapped;
for (i = 0; i < n - 1; i++)
{
swapped = false;
for (j = 0; j < n - i - 1; j++)
{
if (arr[j] > arr[j + 1])
{
// swap arr[j] and arr[j+1]
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = true;
}
}
// IF no two elements were
// swapped by inner loop, then break
if (swapped == false)
break;
}
}
// Function to print an array
static void printArray(int arr[], int size)
{
int i;
for (i = 0; i < size; i++)
System.out.print(arr[i] + " ");
System.out.println();
}
// Driver program
public static void main(String args[])
{
int arr[] = { 64, 34, 25, 12, 22, 11, 90 };
int n = arr.length;
bubbleSort(arr, n);
System.out.println("Sorted array: ");
printArray(arr, n);
}
}
Trên Python3
# Python3 Optimized implementation
# of Bubble sort
# An optimized version of Bubble Sort
def bubbleSort(arr):
n = len(arr)
# Traverse through all array elements
for i in range(n):
swapped = False
# Last i elements are already
# in place
for j in range(0, n-i-1):
# traverse the array from 0 to
# n-i-1. Swap if the element
# found is greater than the
# next element
if arr[j] > arr[j+1] :
arr[j], arr[j+1] = arr[j+1], arr[j]
swapped = True
# IF no two elements were swapped
# by inner loop, then break
if swapped == False:
break
# Driver code to test above
arr = [64, 34, 25, 12, 22, 11, 90]
bubbleSort(arr)
print ("Sorted array :")
for i in range(len(arr)):
print ("%d" %arr[i],end=" ")
Trên C#
// Optimized C# implementation
// of Bubble sort
using System;
class GFG
{
// An optimized version of Bubble Sort
static void bubbleSort(int []arr, int n)
{
int i, j, temp;
bool swapped;
for (i = 0; i < n - 1; i++)
{
swapped = false;
for (j = 0; j < n - i - 1; j++)
{
if (arr[j] > arr[j + 1])
{
// swap arr[j] and arr[j+1]
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = true;
}
}
// IF no two elements were
// swapped by inner loop, then break
if (swapped == false)
break;
}
}
// Function to print an array
static void printArray(int []arr, int size)
{
int i;
for (i = 0; i < size; i++)
Console.Write(arr[i] + " ");
Console.WriteLine();
}
// Driver method
public static void Main()
{
int []arr = {64, 34, 25, 12, 22, 11, 90};
int n = arr.Length;
bubbleSort(arr,n);
Console.WriteLine("Sorted array");
printArray(arr,n);
}
}
Trên PHP
<?php
// PHP Optimized implementation
// of Bubble sort
// An optimized version of Bubble Sort
function bubbleSort(&$arr)
{
$n = sizeof($arr);
// Traverse through all array elements
for($i = 0; $i < $n; $i++)
{
$swapped = False;
// Last i elements are already
// in place
for ($j = 0; $j < $n - $i - 1; $j++)
{
// traverse the array from 0 to
// n-i-1. Swap if the element
// found is greater than the
// next element
if ($arr[$j] > $arr[$j+1])
{
$t = $arr[$j];
$arr[$j] = $arr[$j+1];
$arr[$j+1] = $t;
$swapped = True;
}
}
// IF no two elements were swapped
// by inner loop, then break
if ($swapped == False)
break;
}
}
// Driver code to test above
$arr = array(64, 34, 25, 12, 22, 11, 90);
$len = sizeof($arr);
bubbleSort($arr);
echo "Sorted array : \n";
for($i = 0; $i < $len; $i++)
echo $arr[$i]." ";
?>
Kết quả:
Sorted array:
11 12 22 25 34 64 90
3. Độ phức tạp
Độ phức tạp thời gian của trường hợp xấu nhất và trung bình: O (n * n). Trường hợp xấu nhất xảy ra khi mảng được sắp xếp ngược lại.
Độ phức tạp về thời gian của trường hợp tốt nhất: O (n). Trường hợp tốt nhất xảy ra khi mảng đã được sắp xếp.
Không gian phụ trợ: O (1)
Trường hợp ranh giới: Sắp xếp bong bóng mất thời gian tối thiểu (Thứ tự của n) khi các phần tử đã được sắp xếp.
Sắp xếp tại chỗ: Có
Ổn định: Có
Nguồn và Tài liệu tiếng anh tham khảo:
Tài liệu từ cafedev:
- Full series tự học Cấu trúc dữ liệu và giải thuật từ cơ bản tới nâng cao tại đây nha.
- Ebook về Cấu trúc dữ liệu và giải thuật tại đây.
- Các series tự học lập trình khác
Nếu bạn thấy hay và hữu ích, bạn có thể tham gia các kênh sau của cafedev để nhận được nhiều hơn nữa:
Chào thân ái và quyết thắng!